-
Notifications
You must be signed in to change notification settings - Fork 10
/
setup.py
199 lines (157 loc) · 6.47 KB
/
setup.py
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
# encoding: utf-8
"""
This is a hack allowing you installing
uWSGI and uwsgidecorators via pip and easy_install
since 1.9.11 it automatically detects pypy
"""
import os
import sys
import errno
import shlex
import uwsgiconfig
from setuptools import setup
from setuptools.command.build_ext import build_ext
from distutils.core import Extension
class uWSGIBuildExt(build_ext):
UWSGI_NAME = 'pyuwsgi'
UWSGI_PLUGIN = 'pyuwsgi'
UWSGI_PROFILE = 'pyuwsgi'
def build_extensions(self):
self.uwsgi_setup()
# XXX: needs uwsgiconfig fix
self.uwsgi_build()
if 'UWSGI_USE_DISTUTILS' not in os.environ:
# XXX: needs uwsgiconfig fix
# uwsgiconfig.build_uwsgi(self.uwsgi_config)
return
else:
# XXX: needs uwsgiconfig fix
os.unlink(self.uwsgi_config.get('bin_name'))
# FIXME: else build fails :(
for baddie in set(self.compiler.compiler_so) & set(('-Wstrict-prototypes',)):
self.compiler.compiler_so.remove(baddie)
build_ext.build_extensions(self)
def uwsgi_setup(self):
profile = os.environ.get('UWSGI_PROFILE') or 'buildconf/%s.ini' % self.UWSGI_PROFILE
if not profile.endswith('.ini'):
profile = profile + '.ini'
if '/' not in profile:
profile = 'buildconf/' + profile
# FIXME: update uwsgiconfig to properly set _EVERYTHING_!
config = uwsgiconfig.uConf(profile)
# insert in the beginning so UWSGI_PYTHON_NOLIB is exported
# before the python plugin compiles
ep = config.get('embedded_plugins').split(',')
if self.UWSGI_PLUGIN in ep:
ep.remove(self.UWSGI_PLUGIN)
ep.insert(0, self.UWSGI_PLUGIN)
config.set('embedded_plugins', ','.join(ep))
config.set('as_shared_library', 'true')
config.set('bin_name', self.get_ext_fullpath(self.UWSGI_NAME))
try:
os.makedirs(os.path.dirname(config.get('bin_name')))
except OSError as e:
if e.errno != errno.EEXIST:
raise
self.uwsgi_profile = profile
self.uwsgi_config = config
def uwsgi_build(self):
uwsgiconfig.build_uwsgi(self.uwsgi_config)
# XXX: merge uwsgi_setup (see other comments)
for ext in self.extensions:
if ext.name == self.UWSGI_NAME:
ext.sources = [s + '.c' for s in self.uwsgi_config.gcc_list]
ext.library_dirs = self.uwsgi_config.include_path[:]
ext.libraries = list()
ext.extra_compile_args = list()
for x in uwsgiconfig.uniq_warnings(
self.uwsgi_config.ldflags + self.uwsgi_config.libs,
):
for y in shlex.split(x):
if y.startswith('-l'):
ext.libraries.append(y[2:])
elif y.startswith('-L'):
ext.library_dirs.append(y[2:])
for x in self.uwsgi_config.cflags:
for y in shlex.split(x):
if y:
ext.extra_compile_args.append(y)
LONG_DESCRIPTION = """
# The uWSGI server as a Python module
## Install
```
pip install pyuwsgi
```
## Run
uWSGI will get installed to your Python path with a console script named `pyuwsgi`. To
make it a full drop-in replacement it will install a script named `uwsgi` as well.
You can also call it directly in your Python code with a list of valid uWSGI options:
```python
import pyuwsgi
pyuwsgi.run(["--help"])
```
## Differences from uWSGI
This is built from uWSGI's source without any modifications.
A different [`setup.py`](https://github.com/unbit/uwsgi/blob/uwsgi-2.0/setup.pyuwsgi.py)
is used to make the project a friendlier part of the Python ecosystem. It allows it
to be imported as a Python module and distributed using the
[wheel format](https://www.python.org/dev/peps/pep-0427/). The pre-packaged wheels
include the following common libraries used by uWSGI:
* [zlib](https://zlib.net/)
* [pcre](https://www.pcre.org/)
* [jansson](http://www.digip.org/jansson/)
SSL is intentionally excluded for security reasons. If you need SSL support, you can
force a wheel to be built locally with the `pip` flag `--no-binary=pyuwsgi`.
In addition to the default plugins, the [`stats_pusher_statsd`](https://uwsgi-docs.readthedocs.io/en/latest/Metrics.html#statsd)
plugin is included by default in `pyuwsgi` where it is typically optional for uWSGI.
The full uWSGI documentation can be found at
[https://uwsgi-docs.readthedocs.io](https://uwsgi-docs.readthedocs.io).
## License
uWSGI is licensed [GPLv2](https://www.gnu.org/licenses/old-licenses/gpl-2.0.txt) with
a [linking exception](https://en.wikipedia.org/wiki/GPL_linking_exception) which means
you are allowed to use uWSGI (or pyuwsgi) unmodified in a proprietary or otherwise non-GPL
licensed project without invoking the GPL on the rest of the code.
The [full license](https://github.com/unbit/uwsgi/blob/uwsgi-2.0/LICENSE) can be found
on GitHub.
---
[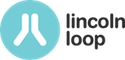](https://lincolnloop.com)
`pyuwsgi` is sponsored by [Lincoln Loop](https://lincolnloop.com).
[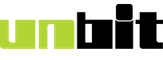](http://unbit.com/)
`uwsgi` is the creation of [Unbit](http://unbit.com/).
"""
setup(
name='pyuwsgi',
license='GPL2',
version=uwsgiconfig.uwsgi_version + "",
author='Unbit',
author_email='info@unbit.it',
description='The uWSGI server',
url='https://uwsgi-docs.readthedocs.io/en/latest/',
long_description=LONG_DESCRIPTION,
long_description_content_type="text/markdown",
cmdclass={
'build_ext': uWSGIBuildExt,
},
py_modules=[
'uwsgidecorators',
],
ext_modules=[
Extension('pyuwsgi', sources=[]),
],
entry_points={
'console_scripts': ['pyuwsgi=pyuwsgi:run', 'uwsgi=pyuwsgi:run'],
},
classifiers=[
'Development Status :: 5 - Production/Stable',
"Environment :: Web Environment",
"License :: OSI Approved :: GNU General Public License v2 (GPLv2)",
"Operating System :: MacOS :: MacOS X",
"Operating System :: POSIX",
"Programming Language :: Python",
"Programming Language :: Python :: 3",
"Topic :: Internet :: WWW/HTTP",
"Topic :: Internet :: WWW/HTTP :: WSGI",
"Topic :: Internet :: WWW/HTTP :: WSGI :: Server",
"Topic :: Internet :: WWW/HTTP :: Dynamic Content",
]
)